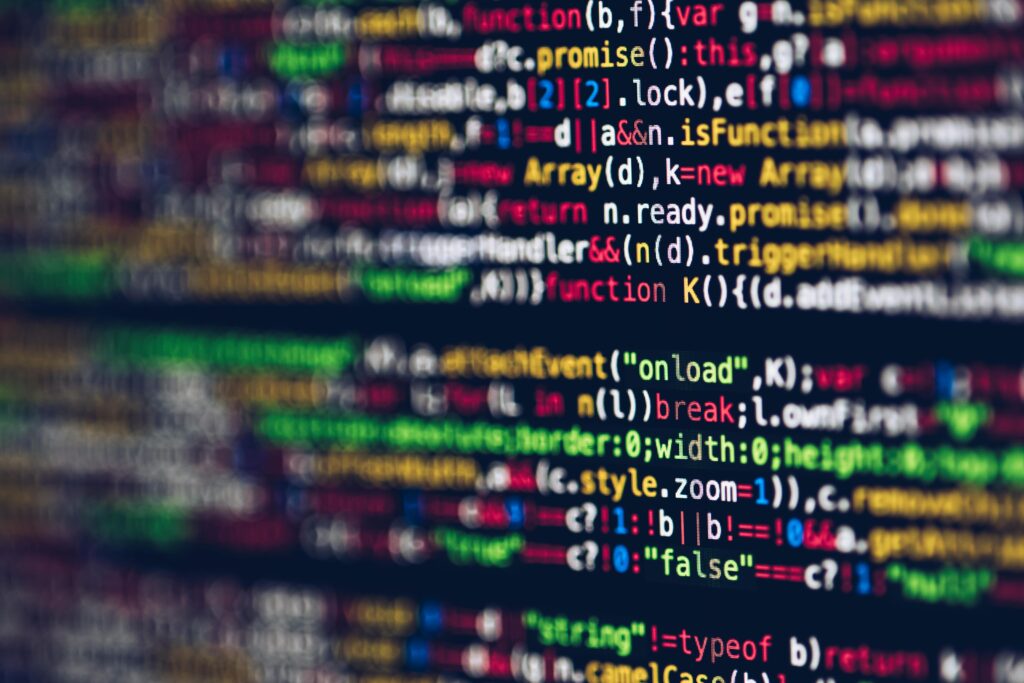
Basic SQL Query Interview Questions
Overview of Basic SQL Query Interview Questions
Basic SQL query interview questions typically focus on fundamental SQL concepts, such as retrieving data from a single table, filtering rows using WHERE clause, sorting data, and using aggregate functions. These questions assess candidates’ understanding of SQL syntax and basic data manipulation techniques.
- What is SQL and its significance in database management? SQL (Structured Query Language) is a standard programming language used for managing and manipulating relational databases. It allows users to query and manipulate data stored in a database, making it essential for database management and data analysis tasks.
- Explain the difference between SQL and MySQL. SQL is a standard programming language used for managing relational databases, while MySQL is an open-source relational database management system (RDBMS) that uses SQL as its query language. MySQL is one of several database management systems that support SQL.
- How do you retrieve all records from a table? To retrieve all records from a table, you can use the SELECT statement without any conditions, as follows:sqlCopy code
SELECT * FROM table_name;
This query selects all columns (*) from the specified table_name. - What is the purpose of the WHERE clause in SQL queries? The WHERE clause is used to filter rows returned by a SQL query based on specified conditions. It allows you to specify criteria that must be met for a row to be included in the result set. For example:sqlCopy code
SELECT * FROM table_name WHERE condition;
This query selects all rows from the table_name where the specified condition is true. - How do you sort data in SQL? To sort data in SQL, you can use the ORDER BY clause followed by the column(s) you want to sort by. For example:sqlCopy code
SELECT * FROM table_name ORDER BY column_name;
This query sorts the result set in ascending order based on the values in the specified column_name. You can also specify multiple columns and sort orders (ASC for ascending, DESC for descending). - What are aggregate functions in SQL? Provide examples. Aggregate functions in SQL perform calculations on sets of values and return a single result. Examples of aggregate functions include COUNT(), SUM(), AVG(), MIN(), and MAX(). For example:sqlCopy code
SELECT COUNT(*) AS total_records FROM table_name;
This query calculates the total number of records in the table_name. - How do you eliminate duplicate rows from a result set? To eliminate duplicate rows from a result set, you can use the DISTINCT keyword in conjunction with the SELECT statement. For example:sqlCopy code
SELECT DISTINCT column_name FROM table_name;
This query selects unique values from the specified column_name in the table_name, removing any duplicate rows. - Explain the difference between the DISTINCT and GROUP BY clauses. The DISTINCT clause is used to select unique values from a single column or combination of columns in the result set. It removes duplicate rows from the output. The GROUP BY clause is used to group rows that have the same values into summary rows, often used with aggregate functions to perform calculations on each group.
- What is the difference between INNER JOIN and OUTER JOIN? INNER JOIN retrieves records from both tables that have matching values in the specified columns, based on the join condition. It returns only the rows where there is a match in both tables. OUTER JOIN retrieves records from both tables, even if there are no matching values in the specified columns. It includes rows from one or both tables where there is no match.
Intermediate SQL Query Interview Questions
Overview of Intermediate SQL Query Interview Questions
Intermediate SQL query interview questions delve deeper into SQL concepts, including joins, subqueries, data manipulation language (DML) statements, and data definition language (DDL) statements. These questions assess candidates’ ability to write complex SQL queries, optimize performance, and manipulate database structures.
- Explain the concept of SQL joins. Provide examples of different types of joins. SQL joins are used to combine rows from two or more tables based on a related column between them. Common types of joins include INNER JOIN, LEFT JOIN (or LEFT OUTER JOIN), RIGHT JOIN (or RIGHT OUTER JOIN), and FULL JOIN (or FULL OUTER JOIN). For example:sqlCopy code
SELECT * FROM table1 INNER JOIN table2 ON table1.column_name = table2.column_name;
This query performs an INNER JOIN between table1 and table2 based on the specified column_name. - How do you use subqueries in SQL? Provide examples. Subqueries, also known as nested queries, are queries nested within another query. They can be used to retrieve data from one or more tables based on conditions specified in the subquery. For example:sqlCopy code
SELECT * FROM table_name WHERE column_name IN (SELECT column_name FROM another_table);
This query selects all rows from table_name where the value in column_name matches any value returned by the subquery. - What are the advantages and disadvantages of using subqueries? Advantages of using subqueries include their ability to simplify complex queries, improve query readability, and enable dynamic filtering based on results of inner queries. Disadvantages include potential performance issues, readability challenges with deeply nested queries, and limitations on their use in certain contexts.
- How do you perform data modification using SQL’s DML statements? SQL’s Data Manipulation Language (DML) statements are used to insert, update, and delete data in a database. Common DML statements include INSERT, UPDATE, and DELETE. For example:sqlCopy code
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
This query inserts a new row into table_name with the specified values for column1 and column2. - What are the differences between DELETE, TRUNCATE, and DROP commands? The DELETE command is used to remove one or more rows from a table based on specified conditions, while the TRUNCATE command is used to remove all rows from a table, resetting the table’s storage space. The DROP command is used to remove an entire table from the database, including its structure and data.
- How do you create and modify database objects using SQL’s DDL statements? SQL’s Data Definition Language (DDL) statements are used to create, modify, and delete database objects such as tables, indexes, and views. Common DDL statements include CREATE, ALTER, and DROP. For example:sqlCopy code
CREATE TABLE table_name (column1 datatype1, column2 datatype2);
This query creates a new table with the specified columns and data types. - What is the purpose of the HAVING clause in SQL queries? Provide examples. The HAVING clause is used to filter rows returned by a GROUP BY clause based on specified conditions. It is similar to the WHERE clause but operates on grouped rows rather than individual rows. For example:sqlCopy code
SELECT column_name, COUNT(*) FROM table_name GROUP BY column_name HAVING COUNT(*) > 1;
This query selects column_name and the count of rows grouped by column_name, only including groups with more than one row. - How do you use SQL functions to manipulate strings, dates, and numeric values? SQL functions are built-in functions that perform operations on data values, such as mathematical calculations, string manipulation, and date/time formatting. Common SQL functions include CONCAT(), SUBSTRING(), DATE_FORMAT(), and ROUND(). For example:sqlCopy code
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM employees;
This query concatenates the values of first_name and last_name columns, returning the result as full_name. - Explain the concept of indexing in SQL and its significance for query performance. Indexing in SQL involves creating data structures that improve the speed of data retrieval operations, such as SELECT queries. Indexes are used to quickly locate rows in a table based on the values of one or more columns. By creating indexes on frequently queried columns, you can reduce the time and resources required to execute SQL queries, resulting in improved query performance.
Advanced SQL Query Interview Questions
Overview of Advanced SQL Query Interview Questions
Advanced SQL query interview questions challenge candidates’ proficiency in writing complex queries, optimizing performance, and solving real-world database problems. These questions may cover topics such as window functions, recursive queries, common table expressions (CTEs), and database normalization.
- How do you use window functions in SQL? Provide examples. Window functions are used to perform calculations across a set of rows related to the current row, without grouping the rows into a single output row. Common window functions include ROW_NUMBER(), RANK(), and NTILE(). For example:sqlCopy code
SELECT employee_id, salary, RANK() OVER (ORDER BY salary DESC) AS rank FROM employees;
This query calculates the rank of each employee based on their salary, using the RANK() window function. - What are recursive queries, and when are they used? Recursive queries, also known as hierarchical queries, are SQL queries that reference their own output. They are used to query hierarchical or tree-like data structures, such as organizational charts or bill-of-materials tables. Recursive queries use common table expressions (CTEs) and the WITH RECURSIVE keyword to define recursive logic.
- Explain the concept of common table expressions (CTEs) in SQL. Common table expressions (CTEs) are temporary result sets that can be referenced within a SELECT, INSERT, UPDATE, or DELETE statement. They provide a way to define complex queries in a modular and readable manner, making it easier to write and maintain SQL code. CTEs are defined using the WITH keyword followed by a query.
- How do you implement pivot and unpivot operations in SQL? Pivot and unpivot operations are used to transform data from rows to columns (pivot) or columns to rows (unpivot) in SQL. Pivot operations involve aggregating and rotating data to generate summary reports, while unpivot operations involve normalizing data into a tabular format. These operations are typically performed using conditional aggregation or CASE expressions.
- What strategies do you use to optimize SQL query performance? Optimizing SQL query performance involves various strategies, including indexing, query optimization, database schema design, and hardware optimization. Common optimization techniques include creating indexes on frequently queried columns, optimizing SQL queries by analyzing execution plans, denormalizing tables to reduce joins, and tuning database server settings.
- What is database normalization, and why is it important? Database normalization is the process of organizing data in a relational database to reduce redundancy and dependency, improve data integrity, and optimize storage efficiency. It involves dividing large tables into smaller tables and defining relationships between them using foreign keys. Normalization ensures that data is structured logically and efficiently, making it easier to maintain and update.
- How do you handle NULL values in SQL queries? NULL values represent missing or unknown data in SQL. To handle NULL values in SQL queries, you can use functions such as IS NULL, IS NOT NULL, COALESCE, and CASE statements. These functions allow you to check for NULL values, replace them with default values, or handle them conditionally based on specific criteria.
Frequently Asked Questions (FAQs)
- What is SQL and why is it important? SQL (Structured Query Language) is a standard programming language used for managing and manipulating relational databases. It allows users to query and manipulate data stored in a database, making it essential for database management and data analysis tasks.
- How can I prepare for a SQL query interview? To prepare for a SQL query interview, review fundamental SQL concepts, practice writing SQL queries, and familiarize yourself with common interview questions. UpskillYourself offers comprehensive SQL courses and practice exercises to help you sharpen your SQL query skills and prepare for interviews effectively.
- Are there any common mistakes to avoid during a SQL query interview? Common mistakes to avoid during a SQL query interview include syntax errors, inefficient query writing, lack of understanding of SQL concepts, and failure to communicate effectively with the interviewer. Practice writing clean and optimized SQL queries to minimize errors and demonstrate your proficiency.
- How can UpskillYourself courses help in mastering SQL query skills? UpskillYourself offers a wide range of AI and cloud courses, including comprehensive SQL courses designed to help learners master SQL query skills. Our expert-led courses cover basic to advanced SQL concepts, provide hands-on practice exercises, and offer real-world examples to enhance your SQL proficiency and prepare you for success in SQL query interviews and database management roles. With our interactive learning platform and personalized support, you can develop the skills and confidence needed to excel in the field of SQL and database management.